🎧 Java Interview Questions
Java is one of the most important object-oriented programming languages today. Prepare yourself with insights on Java interview questions from industry experts in this article below, made more realistic with a detailed success story from Dublin. We want you to be ready with all the topics we have mentioned here.
Read more: How to Prepare for TCS NQT Aptitude? »
Java Objects and Classes
One of the most important concepts of Java interview for the TCS Ninja exam is related to objects and classes. Even after I learned to use them, I practiced a lot to learn about most of the common best practices to use them efficiently. Java includes most of the features of an object oriented programming language. These include polymorphism, inheritance, encapsulation, abstraction, instance, method, and message passing. I realized it is important to clearly understand the difference between an object and a class. A class can be defined as the template of an object. Another word that can be used beside template is blueprint. So basically a class defines all the attributes that the different objects belonging to that class will contain. So it is important to remember to create a class holistically and covering all the possible blueprints. I found this concept of class very interesting as it made me provide some amount of flexibility to the programs. A class can contain variables. Variables can be of a local variable type, or an instance variable type, or a class variable type. It is important to understand each of these variables. A local variable is defined inside a constructor, or a block, or a method. This type of variable is destroyed when the method is completed. The scope of an instance variable is inside the class, but outside any methods. Instance variables are by default initialized when the class is instantiated. They can be accessed by any method, or a block, or a constructor inside that class. Finally, class variables are variables that are declared within a class with the static keyword.
Keep reading: How to Prepare for TCS Digital Aptitude? »
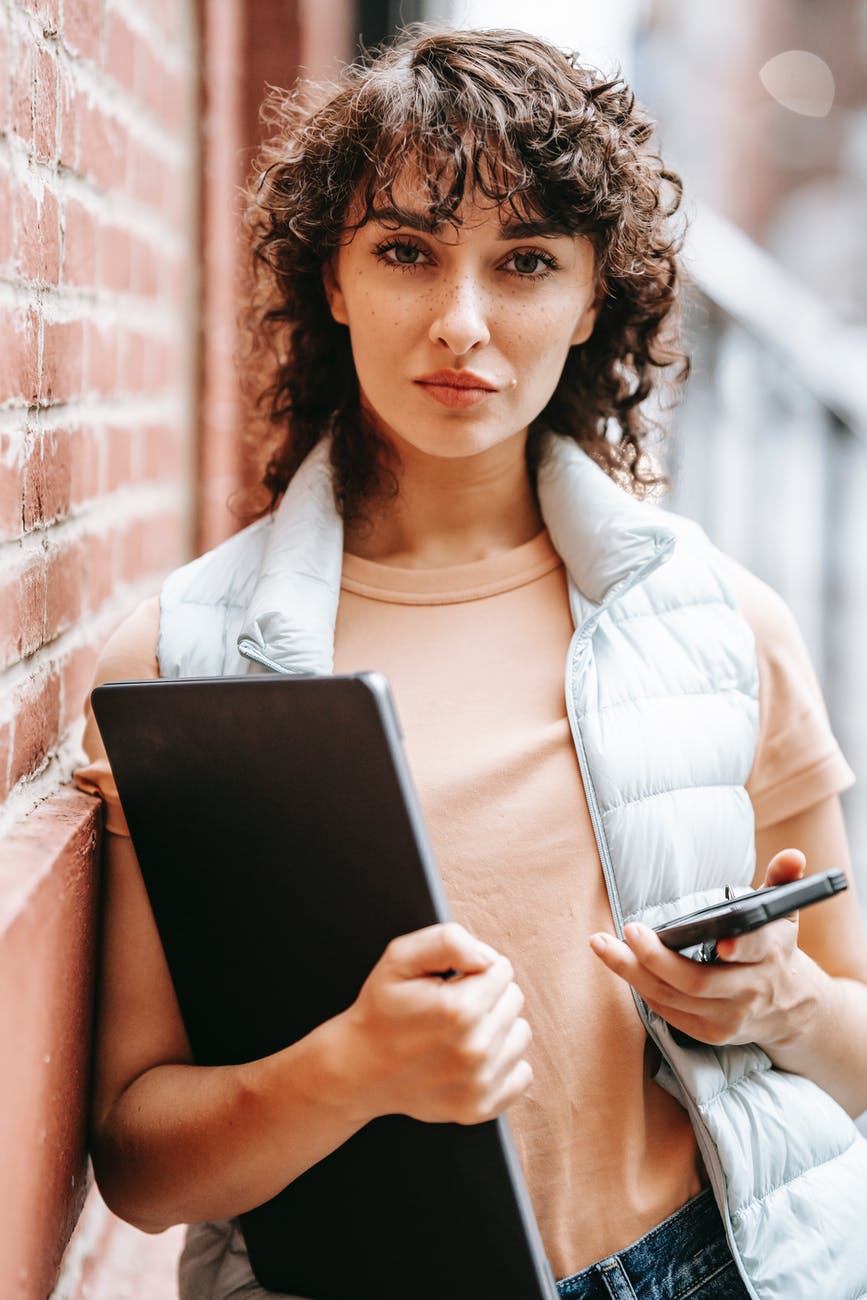
Java Constructors
One important topic for the TCS Ninja exam is constructors. By default, the Java compiler will build a default constructor for any class where we do not define a constructor explicitly. If we define our own constructor, in that case Java will not build a default constructor. A constructor is involved every time that a new object is created. One of the main important rules of a constructor that I learned is that they should have the same name as the name of the class. It is also important to note that a class can have more than one constructor. As I practiced various different Java programs, I came to be familiar with most of these concepts. The constructor I found is one of the most important concepts that I needed to be absolutely clear about. The TCS Ninja exam and the following interviews required me to be absolutely comfortable and crystal clear with these concepts. How does a default constructor work? What are the different types of variables that I can create in a class? What is a class in Java? Did I clearly understand all the features of an object oriented programming language? Did I clearly understand all the scope of the different variables and methods? These were some of the most important things that I wanted to be aware of, and be ready for the main exam. Having good knowledge of object oriented programming in Java is essential for the TCS Ninja exam.
Be TCS ready @ $1.3 per month.
🎁 Did you know you can get $30 for sharing your exam and interview experiences? Write and get paid for every article. Learn more »
The Java Package
An important topic for the TCS Ninja exam is a Java package. A Java package helps us in categorizing the classes and interfaces. I realized this when the size of my programs grew larger. During developing an application, the volume of code written is huge. By categorizing these classes, it will be easier to maintain them. The attributes of scalability and maintainability are extremely important characteristics of a software program. In Java, we can achieve this kind of scalability by using packages. We can use the import statement in Java to include a package in our code. If we give a fully qualified name which includes a package and the class name, then we will help the compiler locate the class. I realized how Java is such a powerful language. We have all the tools we need to implement a high level of flexibility. I started implementing various use cases and wrote my own programs. I used packages and named them as per the best practices whenever possible. I realized that I should be able to do this kind of programs and coding under pressure situations. As I practiced more, I made myself more comfortable with the features of Java packages. I started to love developing Java applications. With the concepts of object oriented programming, I implemented most of the prominent features of Java in my codes. I knew that I need to be able to code efficiently in Java for the TCS Ninja exam.
Keep on reading: C Interview Questions »
Polymorphism in Java
One of the most important features in Java interview programming questions I learnt is polymorphism. Polymorphism means the ability of something to take various forms. With lots of practice I came to realize that the most common use of polymorphism in object oriented programming happens when a particular parent class reference is used to refer to a child class object. The most important fact that we should be aware regarding polymorphism is that there is only one way that we can access an object. We can access an object using a reference variable. Now, a reference variable can be of only one particular type. Also, the type of a reference variable cannot be changed once it is declared. The reference variable can be reassigned to other objects as long as it is not declared as a final reference variable. Type of the reference variable is also important. Since the type will determine the methods that the reference variable can invoke on the object. I recognized these key concepts as crucial for my strong understanding of Java. Also, a reference variable is able to refer to any object which is of its declared type, or of any sub type of its declared type. I wanted to be good in Java programming. I wanted to implement the concept of polymorphism wherever I could. I also understood that a difference variable can only be declared as a class type or an interface type. These concepts were very crucial for me for the TCS Ninja exam.
Method Overriding in Java
I started to understand how overridden methods in Java allows us to utilize polymorphism to its full potential. In method overriding, I knew that a child class can override a method in its parent class. And it is also essential to remember that an overridden method is actually hidden in the parent class. It is not invoked unless our child class uses the keyword called super within the overriding method. An interesting concept I wanted to implement and practiced a lot is virtual method invocation. In this context, these methods are referred to as virtual methods. I practiced a lot of these programs so that I became fully aware of these concepts. With more practice, any doubt that I had regarding polymorphism became clear to me. It is important to remember that an overridden method is invoked at runtime. It does not matter with what data type the reference was used in the source code at compile time. Questions on polymorphism are extremely important for a Java interview. While preparing for the TCS Ninja examination, I wanted to be a good Java programmer. I wanted to be able to answer all the questions from Java. If the interviewer asked me. Even for the Hanson and coding sections, I wanted to be able to write good and efficient Java programs. Sometimes it is not only enough to be able to write a program, but also if the program is written optimally following the best practices.
Abstraction in Java
Abstraction is an important feature in Java interview. It is one of the key features in Java as an object oriented programming language that makes it special. What do we mean by abstraction? Abstraction means an idea, something less than specifics. In Java, abstraction is the process of hiding the details of how a logic is implemented from the user. Thus the user will only be able to experience the functionality provided by this logic. Since we are working with objects in Java, this means that the user will see what the object is doing instead of how the object is doing it. I found this an extremely robust feature in a programming language. Abstract is a keyword in Java. A class in which the abstract keyword is present during its declaration is defined as an abstract class. It is important to note that an abstract class may contain an abstract method and also may not contain an abstract method. What is an abstract method? An abstract method is a method without a body. A class must be declared as abstract if it has at least one abstract method. An important feature of an abstract class is that if it is declared abstract, it cannot be instantiated. I learned that I can use an abstract class if I inherit it from another class, and provide the implementation logic details to the abstract methods inside it. I implemented abstract classes in many of my Java interview practice programs so that I learned all the logics behind them and also how to efficiently use them.
Abstract Methods in Java
An important topic for the TCS Ninja exam is abstract method. What are abstract methods in Java? We can get a scenario where we want a class to contain a method, but we want the actual implementation of that method to be determined by the child classes. In such a scenario, we can declare the method in the parent class as an abstract method. I realized that to become familiar with an abstract method, I needed to implement and write programs. I started practicing hard and wrote multiple programs with abstract methods. To declare a method as an abstract method we have to use the abstract keyword. Understanding all the features of abstraction give me a comprehensive understanding of preparing for a Java interview. I came to realize that without abstraction realizing the full potential of Java was impossible. I imagined there can be so many use cases where abstractions can be an extremely helpful feature in Java. We have to place the abstract keyword just before the name of the method during the declaration of the method. The unique feature of an abstract method is that we do not have a method body, but we only have a method signature. Also, it is important to note that instead of curly braces at the end of an abstract method, we will have semi colon. To declare an abstract method, we have to declare the class containing it as abstract. And also, any class inheriting this abstract class must override the abstract method, or otherwise declare itself as an abstract class.
Encapsulation in Java
One of the most important characteristics of Java interview is encapsulation. Encapsulation is an important topic for the TCS Ninja exam. I was surprised at how much features and flexibility Java provided as an object oriented language. Encapsulation is one of the major fundamental object oriented programming concepts. Now let’s understand what is encapsulation? Encapsulation helps the wrapping up of various components like the variables and code which are acting on the methods into a single unit. In this scenario, the variables of a particular class will always be hidden from the other classes. Also, this variable can only be accessed through the methods defined in the immediate current class. In this context, it is also known as data hiding. In order to achieve encapsulation, we have to declare the variables of our class as a private variable. We also have to provide a public set and a get method which will help us to modify and view the variable values when needed. I found it extremely important to remember the benefits of encapsulation. Using encapsulation, we can specify the fields of a class as read-only or write-only. Another feature of encapsulation in Java is that a class can have complete control over what is stored in each of the fields. I made sure that I practiced a lot of Java interview programs using encapsulation. This practice helped me get a clear knowledge and understanding of this important object oriented programming feature.
Inheritance in Java
Inheritance is a key feature of object oriented programming in a Java interview. Inheritance can be defined as the process by which one class can acquire the properties of another class. The class which is inheriting the property from the other class is known as a subclass, or a derived class, or also known as a child class. The class whose properties are inherited is defined as the superclass, or a base class, or also known as the parent class. I wanted to implement inheritance in many of my programs. The keyword I used to inherit the properties of a class is extends. Extends is the most important keyword that we need to remember while incorporating inheritance in Java programming. It is important to remember that a superclass reference variable can hold a subclass object, but if we are using that variable, we can only access the members of the superclass. Hence, to access the members of both the classes, we will have to create a reference variable to the subclass. One of the most important things that I learned is that a subclass will inherit all the fields, the methods, and the nested classes from its superclass. We also have to remember that since constructor’s are not members, they will not be inherited by subclasses. However, we can invoke the constructor of the superclass from a subclass. I tried to implement my new concepts in the codes that I wrote. Inheritance is an important topic for the TCS Ninja exam.
Super keyword in Java
The Super keyword is very important in a Java interview. During my preparation and learning of Java for the TCS Ninja exam, I made sure that I was comfortable with the Super keyword. The Super keyword is very similar to the this keyword in several ways. The Super keyword is most often used to distinguish between the members of the superclass from the members of the subclass. This is especially useful if the members between the superclass and the subclass have the same names. The Super keyword can also be used to invoke the superclass constructor from our subclass. An important feature of inheritance is that if we have a class which is inheriting the properties of another class, then the subclass will acquire the default constructor of the superclass. But in a scenario where we want to call a parameterized constructor of the superclass, we will need to use the super keyword along with the required parameterized values. Instance of operator is helpful to determine whether an object is actually a member of the superclass that we presume it to be. It returns the value true if it is actually an instance of that class. The different types of inheritance that I learnt is single inheritance, multilevel inheritance, hierarchical inheritance, and multiple inheritance. A single inheritance occurs when one class inherits from another class. A multi level inheritance occurs when one class inherits from another class. and another class inherits from that subclass. A hierarchical inheritance occurs when multiple classes inherit from one parent class. Multiple inheritance occurs when a subclass inherits from more than one superclass.
TCS Interview Questions
An unique, live, and continuously updated database of the latest TCS Interview questions.
Get In Touch
Who we are
